If you want to know how to extract Chrome Password in Python then read this article till end. I shared different methods to extract password using python.
Google Chrome, one of the most popular and secure web browsers, stores passwords securely in its database, ensuring easy access for users. However, as a Python programming expert, you can leverage your skills to access this database and retrieve the stored passwords. You can create a script that decrypts and extracts passwords from the Chrome password database by understanding the underlying mechanisms and employing Python’s capabilities.
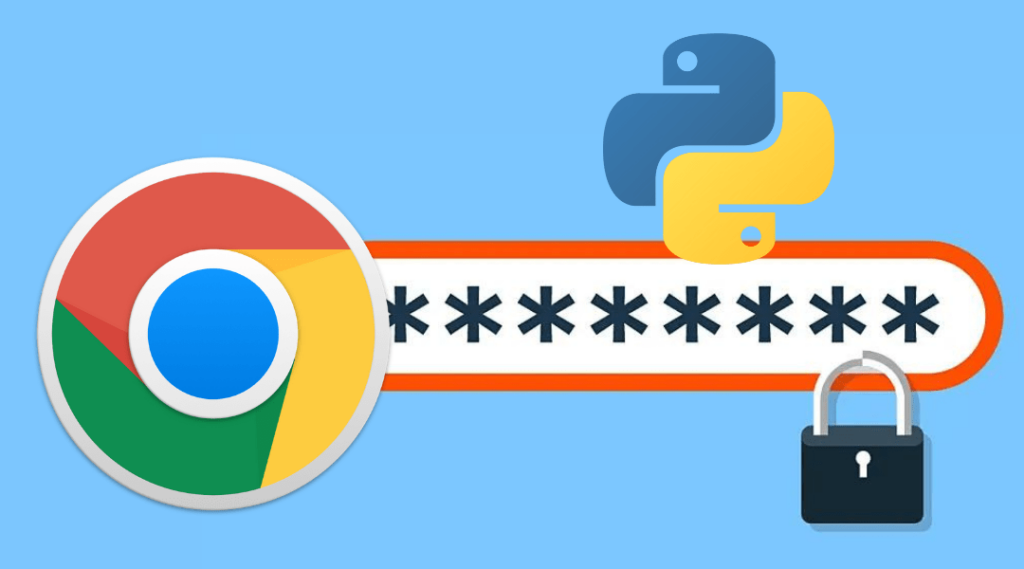
Throughout this blog, we will explore the technical aspects of Chrome password storage, the encryption methods employed by Chrome, and the necessary steps to extract passwords using Python. We will also discuss best practices and security considerations to ensure the responsible and ethical use of the extracted passwords.
Before coding, let’s first understand how google chrome password storage works.
How does Google Chrome store passwords?
Google Chrome’s password storage system, Chrome Password Manager, securely saves and manages website passwords. It encrypts passwords using industry-standard methods and offers synchronisation across devices. There is no dedicated master password feature, relying instead on your operating system’s user account password.
Chrome can autofill passwords and periodically checks for compromised passwords. You can manage your saved passwords through Chrome’s built-in password manager. Additional security measures like two-factor authentication are recommended for enhanced security which helps to make it more secure from hackers attacks.
Let us start developing a python script to extract Chrome passwords in python.
To successfully extract Chrome passwords, it’s crucial to comprehend how Google Chrome stores this sensitive information. Chrome Password Manager securely encrypts and stores passwords using industry-standard encryption algorithms like AES-256.
The passwords are stored locally on the user’s computers, making it imperative to have physical access to the computer to retrieve them. Additionally, Chrome offers a synchronisation feature that allows passwords to be shared across devices if the user is signed in with their Google Account.
Get Started: How to Extract Chrome Password in Python
Before we can embark on our quest to extract Chrome passwords, we need to ensure our arsenal is fully equipped with everything, so we will follow a step-by-step process to write a script.
Setup Environment
It is very much necessary to create a virtual environment for separate Python projects as it meant cause version issues when your work with multiple projects together.
Creating a python virtual environment is very easy, and you can follow the same steps in all the operating systems and follow the below steps.
To create a virtual environment in python, run the next command and your respective operating system terminal:
python -m venv myenv |
Replace “myenv” with the desired name for your virtual environment. Activate the virtual environment using the command for your operating system:
- Windows:
myenv\Scripts\activate |
- macOS/Linux:
source myenv/bin/activate |
With your virtual environment activated, you can proceed to install the required libraries and execute your Python scripts within this isolated environment.
Python offers a vast ecosystem of libraries and packages that extend its capabilities. We must install specific libraries to help us interact with Chrome’s password storage. Open your command prompt or terminal and run the following commands:
pip install sqlite3 |
And
pip install pycryptodome |
Now that you have set up Python and installed the necessary dependencies, you’re ready to venture further into the realm of Chrome password extraction.
Extracting Chrome Passwords with Python
Let’s code step by step how to extract passwords with python.
Step 1: Locating the Chrome Password Database:
We need to locate the Chrome password database file to begin our quest. By default, you can find this file at the following path:
C:\Users\Username\AppData\Local\Google\Chrome\User Data\Default\Login Data
Replace “Username” with your existing operating system username.
Step 2: Connecting to the Database:
Python comes with the sqlite3 library, which allows us to interact with SQLite databases. We will use this library to establish a connection to the Chrome password database. Let’s take a look at the code snippet below:
Example on How to Extract Chrome Password in Python.
import sqlite3 database_path = r’C:\Users\YourUsername\AppData\Local\Google\Chrome\User Data\Default\Login Data’ # Connect to the database conn = sqlite3.connect(database_path) cursor = conn.cursor() |
Make sure to replace YourUsername with your actual username and database_path with the path to your system’s Chrome password database file.
Step 3: Retrieving the Password Data:
Now that we are connected to the database, we can execute SQL queries to retrieve the encrypted passwords along with their associated website URLs and usernames. Here’s an example:
# Execute SQL query to retrieve password data cursor.execute(“SELECT origin_url, username_value, password_value FROM logins”) # Fetch all the results results = cursor.fetchall() # Iterate through the results for row in results: url = row[0] username = row[1] encrypted_password = row[2] # Decrypt the password and perform further actions |
In the code snippet above, we select the columns origin_url, username_value, and password_value from the logins table in the database. The results are then fetched and stored in the results variable. We can iterate through the results and extract the URL, username, and encrypted password for further processing.
Step 4: Decrypting the Passwords:
The passwords stored in Chrome’s database are encrypted for security reasons. To unveil their proper form, we need to decrypt them. The pycryptodome library comes to our rescue once again. Here’s an example of how to decrypt the passwords:
from Cryptodome.Cipher import AES import base64 def decrypt_password(encrypted_password): # Chrome uses the DPAPI (Data Protection API) to encrypt passwords # The encrypted password is base64 encoded encrypted_password = base64.b64decode(encrypted_password) # Key used for encryption is stored in the system’s DPAPI folder # We need to retrieve the key using the `win32crypt` library (Windows-only) import win32crypt key = win32crypt.CryptUnprotectData(encrypted_password, None, None, None, 0)[1] # Decrypt the password cipher = AES.new(key, AES.MODE_GCM, nonce=encrypted_password[3:15]) decrypted_password = cipher.decrypt(encrypted_password[15:]) return decrypted_password.decode(‘utf-8’) |
In the code snippet above, we define a decrypt_password function that inputs the encrypted password. We decode the base64-encoded password and extract the initialisation vector (IV). With the IV and a predefined key, we create an AES cipher. Finally, we decrypt the password and return it as a string.
Conclusion
In this adventure, we’ve explored how to extract Chrome passwords using Python. We can retrieve encrypted passwords by setting up Python, installing dependencies, and connecting to the Chrome password database. With decryption techniques, we can unveil their true form. Remember to handle passwords securely and follow ethical practices. Now, armed with Python skills, go forth and unlock the secrets of Chrome passwords responsibly. Happy coding!