This tutorial will learn about python break and continue statements and use them in loops and conditional statements.
Python break
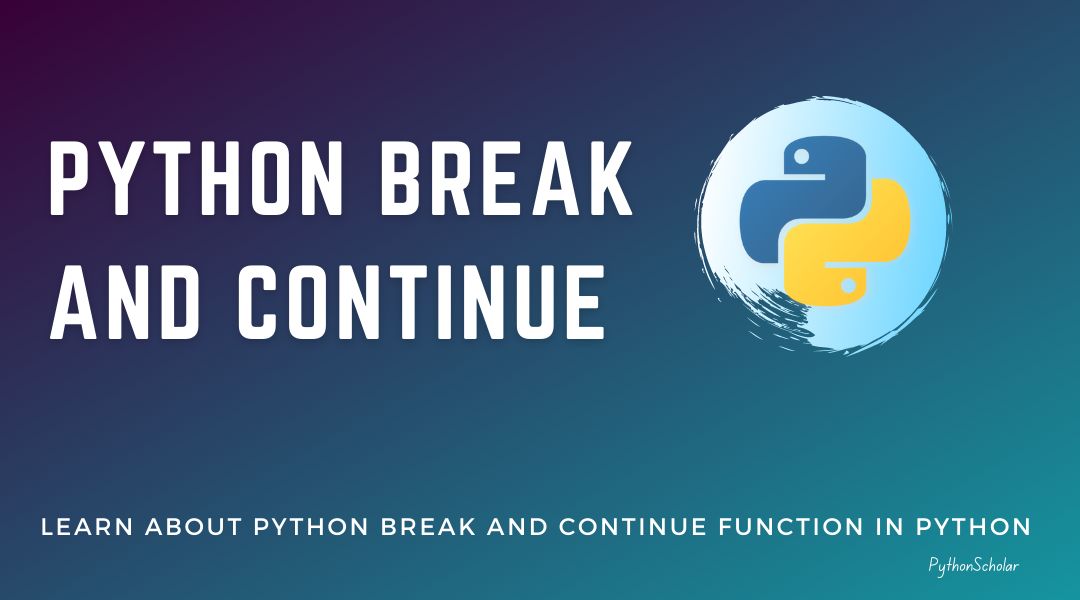
Python break
Break statement in python is used to terminates the current loop and resumes the execution statements. With the break statement, we can stop the loop before it has looped through all the items:
Break Python syntax:
Break statement in for loop
We can use break statements in for loops to exit the current loop, which will terminate the loop’s flow.
Lets see how python break for loop works
Example of the break in python
The output of the break statement program.
Python break statement in if else conditional statements
We can also use the python break function in if else, conditional statement, which will break the conditions’ flow.
Let see an example of a break in conditional statements.
The output will be as follows.
Here the above program will print items in the list one by one. Still, inside the for loop, we have implemented a condition with if the value is banana, then break statement will call which will break the loop, and the flow will be exited so that only one item is printing.
Python continue
The continue statement in python will end the current loop and condition without exiting the main loop; it will end the loop’s current iteration and start the next iteration.
Continue python syntax
Python continue Statement in for loop with if conditional statement.
With the continue statement, we can stop the current iteration of the loop and continue with the next:
Example of python continue in for loop.
The output of the continue statement.
Here we are implementing the if conditional statement inside the for loop, and in the if conditional statement, we are using the continue statement.
The if continental statement is given x equal to banana if the for-loop item will be banana, then if the conditional statement is executed, the continue statement and loop will be skipped so that it is not printing the item banana.
FAQs
What is difference between break and continue in Python?
There is a huge difference between the break and continue statement because break is used to terminate the iterator of the program but on the hand continue will just skip the current loop or iterator.
What is the difference between break and continue in while loop in Python?
In Python, the while loop break statement will break the while loop execution and the continue statement will just skip the current execution.
What is break continue and pass in Python with example?
Here are some examples of Python break and continue pass statements on PythonScholar.
Why do we need break and continue in Python?
Break and continue statements are very important parts of python programming language and these are used with conditional statements, for example, it will break the execution when a certain condition gets fulfilled or it will skip the blow execution when a certain condition is not fulfilled.