In this tutorial, we will learn about Python Tuple and all its methods.
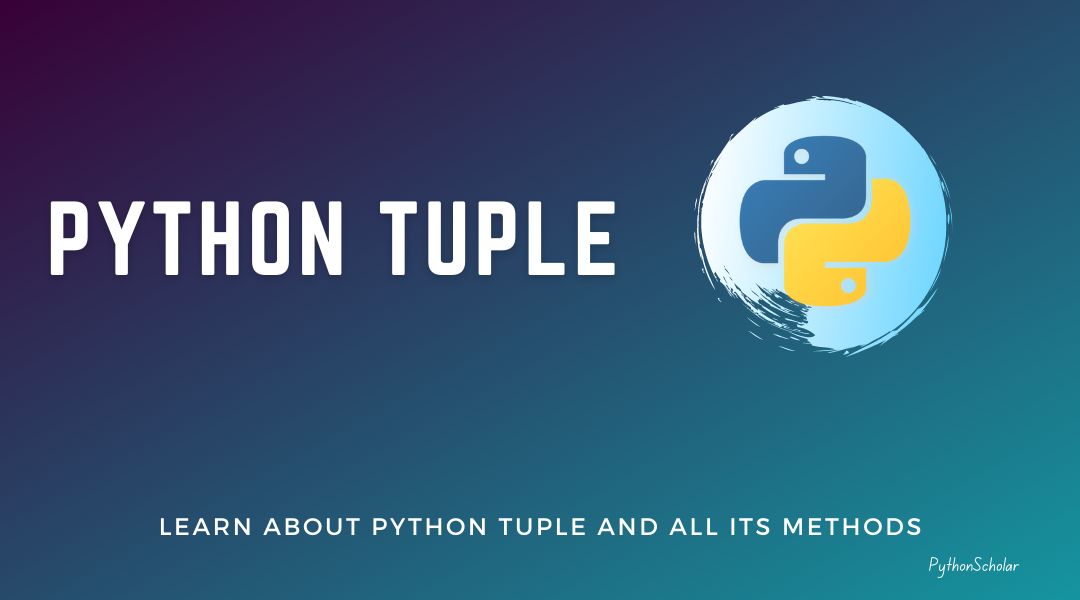
What is python tuple?
A tuple in python is similar to the list in many ways. Like lists, tuples also contain the collection of the items of different data types, and values are separated with a comma (,) but enclosed within parentheses ().
A tuple is immutable, so it is a read-only data structure, and we can’t modify the size and value of the items of a tuple.
A tuple can have any number of items, and they may be of different types (integer, float, list, string, etc.)
Let’s see some of the Python tuple examples below.
Example:
Output:
Now let us check the type function with a tuple.
Example:
Output:
Nested Tuple
We can create a nested tuple by adding a bracket inside the bracket.
Example:
Output:
We can also add a list inside a tuple or add a tuple inside a list.
Let us check examples of how we can add a list inside a tuple.
Example:
When we run this program, we will get the following result:
Output:
Access Tuple Elements
We can access tuple elements by referring to the index number inside square brackets by positive and negative indexes.
Positive Indexing
As we know python index starts from 0, so does it in the tuple.
Example:
Output:
Negative Indexing
Negative indexing means starting from the end like -1 refers to the last item, -2 refers to the second-last item, etc.
Example:
Output:
Slicing of Tuples
We can slice the specific range of indexes by specifying from where to start and where to end the range. We can set range by using a colon: in between the two indexes.
Let’s check an example of slicing tuples in python.
Example:
Here, we will get the result as follow.
Output:
As we can see, it is returning the values of index third, fourth and fifth because the tuple indexes start from 0. That’s why it starts from index2 and ends at index 5, but it does not include 5 because the index has started from 0.
Let us see some more examples of the slicing of tuples.
Example:
Output:
This example returns the items from index -4 (included) to index -1 (excluded)
We can also slice tuples using negative indexing also, let check out.
Example:
When we run, we will get this result.
Output:
Concatenation of Python Tuple
Concatenation to python tuples means to merge or combine two or more tuples into one. We can use the + operator to combine two tuples.
We can also multiple the elements in a tuple to itself for a given number of times using the * operator.
Let’s check an example of the python concatenation of tuples.
Example:
The output will be as follow:
output:
Deleting a Tuple Elements
As we learn that a tuple is immutable, we cannot change elements in a tuple, so also, we cannot delete the elements in a tuple, or we can delete an entire tuple together.
Let we an example
Example:
When we run the following program, we will get.
Output:
But we can delete an entire tuple here.
Example:
Output:
Membership Test in Python Tuple
The membership test is used to find that whether the specific element is present in a tuple or not.
We can do a membership test using whether a conditional statement and also with in keyword to find specific element is present in a tuple or not.
Lets check an example:
Example:
The output will be as follow:
We can also do a membership test without any conditional statement just by using in keyword here.
Example:
Output:
For loop in Python Tuple
We can use for loop to iterate through each item in a tuple.
Example:
When we run this program, we will get the following output:
Tuple Methods
Python has two built-in methods that you can use on tuples.
Method | Description |
count() | Returns the number of times a specified value occurs in a tuple |
index() | Searches the tuple for a specified value and returns the position of where it was found |