In this tutorial, we will learn about all the data types we can use in python.
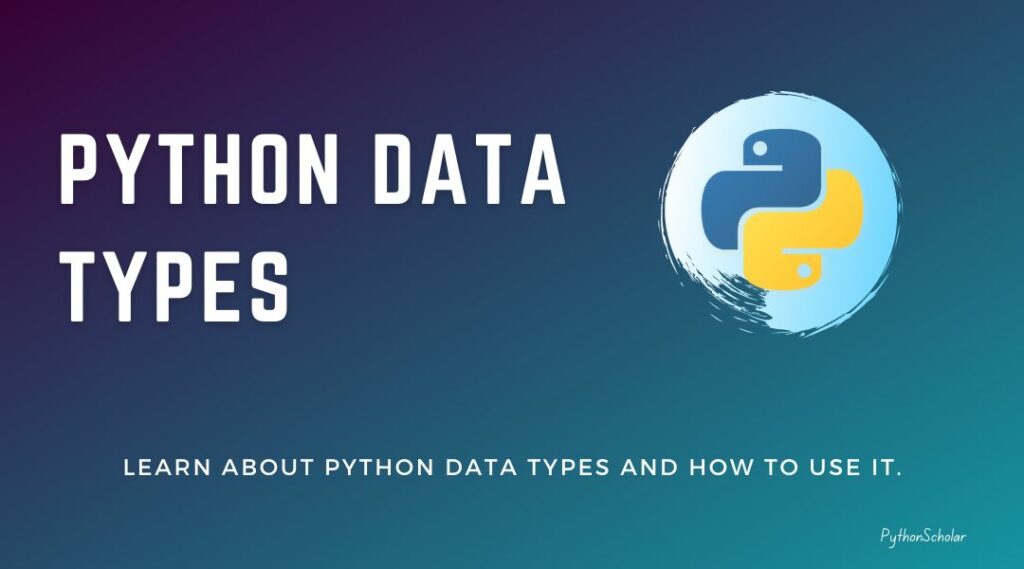
What is data type in python
In all programming languages, data types are the essential concept. Variables use data types to store different types of data that do different things. Unlike other programming languages like C, C++, or JAVA, we do not need to declare data type keywords to declare a variable. In Python, we just need to declare the name of the variable and its value.
Python has the following built-in data types by default.

As we can see in the above diagram, there are nine default python data types in python. All nine data types have different purposes.
Python Text or String Data types
A text or a string data type which can store a value like letters from a-z in the capital as well as in small, Integer values like 0-9 or a float value like 1.5, 3.7, 200.453, etc. and we can also use all the specials characters like @,$,%,&, *. Generally, strings are represented by either single or double quotes.
How to assign string data types.
To implement string, we need to follow the same steps as assigning a variable.
Example:
X = "This is a String Data Type." print(X)
Output:
This is a String Data Type.
Let take another example by using the type() function along with the string data type.
Example:
X = "This is an example of a Type function." print(X) print(type(X))
This is an example of a Type function. <class 'str'>
Learn advanced topics like string slicing, manipulation, and much more from here.
Python Numeric Data Type
Python Numeric data types are used to store numeric values like 0-9, 10000, or like 500.34, 2.4, 0.5, etc.
Follow data types are included in Python Numeric data types:
- int – holds signed integers of non-limited length.
- Long- holds long integers(exists in Python 2.x, deprecated in Python 3.x).
- Float- holds floating precision numbers, and it is accurate up to 15 decimal places.
- Complex- holds complex numbers.
Python int
An int is a data type that stands for integers used to numeric values from 0-9.
Example:
X = 4 Y = 11102144585 print(X) print(Y) print(type(X))
Output:
4 11102144585 <class 'int'>
Python float
This data type is used to store float values like a real number with floating-point representation—examples 1.2, 45.66, 30000.1.
Example:
X = 3.7 print(X) print(type(X))
Output:
3.7 <class 'float'>
Python Complex
As the name suggests, a complex number with a real and an imaginary part like 1+A3 and 7+34i. Unlike other programming languages such as Java or C++, Python can identify these complex numbers with values. Suppose we take an example as 2 + 4j where 2 is a real part and j is the imaginary part.
Here are some examples.
X = 2 + 4j print(X) print(type(X))
Output:
(2+4j) <class 'complex'>
Python Sequence Data Type
Sequential data types are a few of the built-in data structures. Sequential data types are the same as arrays in other programming languages but with advanced features. This is the topic you will often use in your python journey.
Python List
The list is used to store an ordered sequence of values in a single variable, or we can say the list is the collection of the items of different data types. It is a very flexible data type in Python. The list is like an array from the other programming language.
The list is mutable so that we can change the value in the list at any time.
How to Create a List
As a list is a collection of the items, we need to separate all the values with a comma (,), and it should be enclosed in square brackets [ ].
Example:
mylist= [1,2,3,4] print(mylist)
Output:
[1, 2, 3, 4]
We can add any value in the list, but if it is a string, it should be in a single quote (‘) or double quote (“).
Example:
mylist = [1,'two', 3.5, 'four'] print(mylist)
Output:
[1, 'two', 3.5, 'four']
Example:
mylist = [1,'two', 3.5, 'four'] print(type(mylist))
Output:
<class 'list'>
Python Tuple
A tuple is similar to the list in many ways. Like lists, tuples also contain the collection of the items of different data types, and values are separated with a comma (,) but enclosed within parentheses ().
A tuple is immutable, so it is a read-only data structure, and we can’t modify the size and value of the items of a tuple.
Let’s see a example of tuple:
tup = (24 , "Hello", "World", 2021) print(tup)
Output:
(24, 'Hello', 'World', 2021)
Now let us check the type function with a tuple.
tup = (24 , "Hello", "World", 2021) print(type(tup))
Output:
<class 'tuple'>
Python Dict
Python Dictionary is a built-in data structure, and it is also used to store sequential data but in an unordered collection of key and value pairs. Dictionary store values within the curly braces { } and separate with a comma (,).
As Python dictionaries store data in key values, it should be in the following format.
X: 21
Key-value
Example:
mydict = {'X':2, 'Y':3 , 'Z':4} print(mydict)
Output:
{'X': 2, 'Y': 3, 'Z': 4}
Let us take another example of a python dictionary.Values in a dictionary can be of any data type and can be duplicated. Still, the keys can’t be repeated, and we also cannot change the key as python is case sensitive, so dictionaries with the same keys as X and x will be treated distinctly.
House = { 'Door':2, 'Windows':4, 'Garden': 'Yes'} print(House) print(type(House))
Output:
{'Door': 2, 'Windows': 4, 'Garden': 'Yes'} <class 'dict'>
Python Dictionary is a very vast topic. We will learn it in an upcoming tutorial, or you can click here.
Python Set Data Type
Same as Dictionary, Set is also the unordered collection of the items. However, unlike dictionaries in Set, we are not using key-value pairs. In Set, we are just adding values. In Set, all the values are defined inside the curly braces { } and should be separated by comma (,).
Let see some examples of Set Data types.
Example 1:
Myset = { 1, 2, 3, 4, 5} print(Myset)
Output:
{1, 2, 3, 4, 5}
Set is Mutable, so we cannot change the set’s values once we assign it cannot be changed throughout the program.
Example 2:
Set_2 = {'A','B','C','D'} print(Set_2)
Output:
{'A', 'C', 'B', 'D'}
Note: As Set is a collection of unordered items, every time we run set, we will get different ordered output.
Example 3:
Set_2 = {'A','B','C','D'} print(type(Set_2))
Output:
<class 'set'>
Python Boolean
In the Boolean data type, we can just assign two values which are True or False. In Boolean, equal to True is call truthy, and those equal to False are falsy. The first letter should be capital like “T” in True and “F” in False to assign a Boolean value. We do not need to use any single quote or double quote to use Boolean.
Example:
X = True Y = False print(X) print(Y)
Output:
True False
Example 2:
X = True Y = False print(type(X)) print(type(Y))
Output:
<class 'bool'> <class 'bool'>
FAQs
What is compound data types in python
Compound data types in Python are data types that can hold multiple values or data elements. The built-in compound data types in Python are lists, tuples, and dictionaries.
Lists are ordered collections of values or items, which can be of any data type. Lists are mutable, meaning that you can add, remove, or modify items in a list.
Tuples are similar to lists in that they are ordered collections of values, but they are immutable, meaning that you cannot change the values once the tuple is created.
Dictionaries are unordered collections of key-value pairs. Each key in a dictionary must be unique and is associated with a specific value. Dictionaries are mutable, meaning that you can add, remove, or modify key-value pairs.
Compound data types are useful when you need to group related data together, such as a list of names or a dictionary of user information. They provide a convenient way to access and manipulate multiple values at once.
What is the difference between a float and an integer in Python?
A float in Python is a numeric data type that can represent decimal numbers, whereas an integer is a numeric data type that can represent whole numbers only.
How do you check the data type of a variable in Python?
To check the data type of a variable in Python, you can use the type() function. For example, type(42) will return <class ‘int’>.
How do you convert a string to an integer or a float in Python?
To convert a string to an integer in Python, you can use the int() function. For example, int(“42”) will return 42. To convert a string to a float, you can use the float() function. For example, float(“3.14”) will return 3.14.
How do you convert a variable to a string in Python?
To convert a variable to a string in Python, you can use the str() function. For example, str(42) will return “42”.