In this tutorial, we will learn how to execute your first python program on python idle and other OS ; the same steps can be followed using Windows, Linux, and Mac OS.
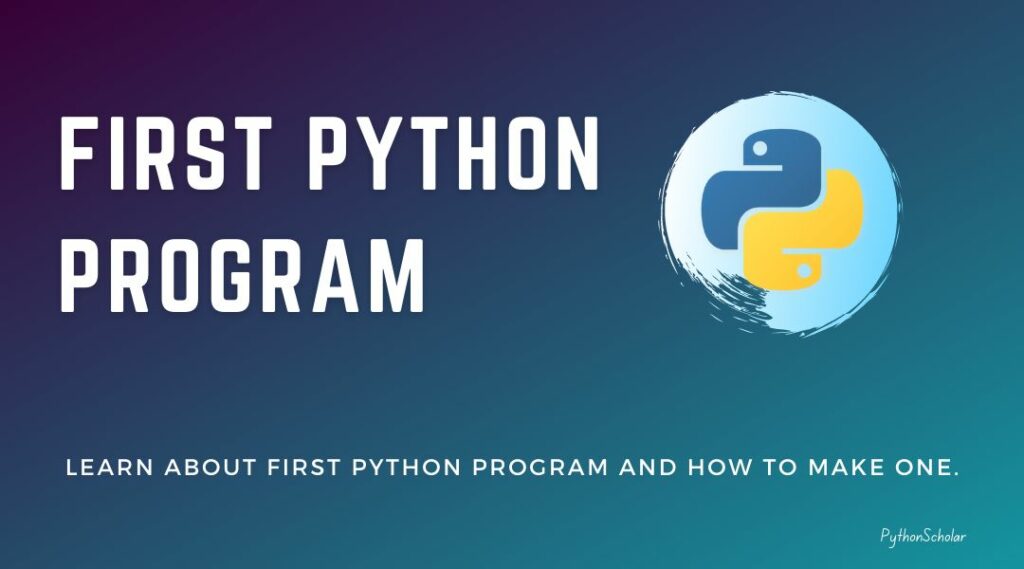
Let us execute our first python program using python idle.
Step 1: Open idle.
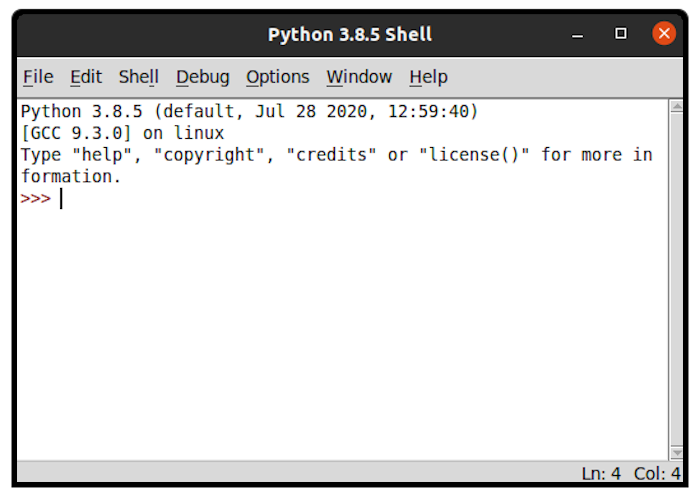
Step 2: Click on File on the top menu and click on New File, or we can just use Keyboard shortcut “Clt + N”
How to Code in Python?
As you see, we have opened the new file on idle now, we will write the python code below and save it by any name using the Clt + S keyboard shortcut, but it should end with the “.py” extension.
Step 3: Let’s begin to code with python by typing the below code.
print(“Hello Python”)
How to Run a Python Program?
Let’s execute our first program by opening the Run menu from the top bar and click on “Run Module” or just the F5 key.
As you see, we have executed our first python program successfully.
Output:
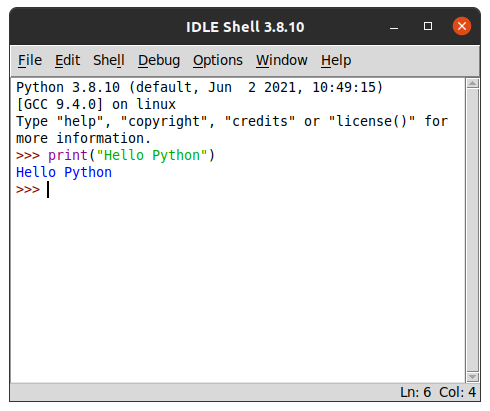
How To Run A Python Program In Windows?
You can use the Windows default command prompt to run the python program in windows. To open CMD(Command prompt), you can type CMD on Run or search CMD in windows start.
Once you open the CMD, you can see a screen like below.
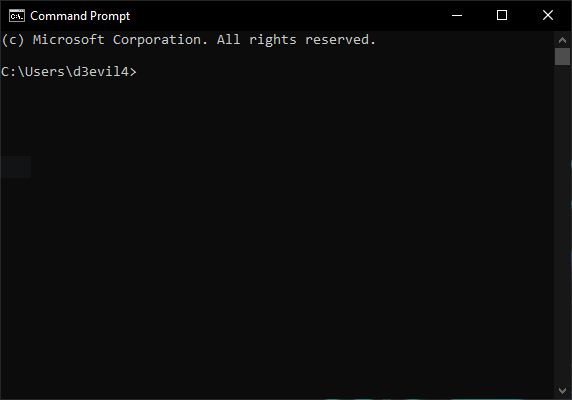
You can type python command to check whether Python is installed or not, and if it is not installed, you can check our Install python page to install python on your respected operating system.
When you type python and press enter, you see the python interpreter, as shown below.
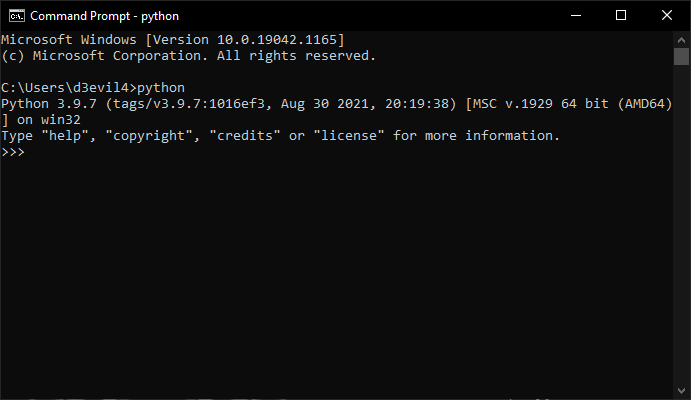
You can type the below code to execute the python program.
print(“Hello World”)
All python programs are stored in a ‘.py’ file, so when we develop any python program, it will be saved in ‘.py’ format.
To run a python program, you can use the python command followed by the file name with the python extension.
python program.py
The output will be as below.
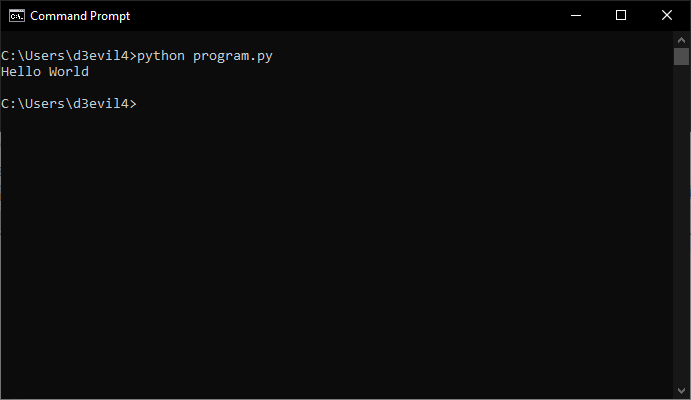
How To Run A Python Program On Linux?
We can use the Linux terminal to run python programs in Linux/Ubuntu, which can be opened by pressing ‘Ctrl + Alt + T’ or just search terminal in the search bar of your operating system.
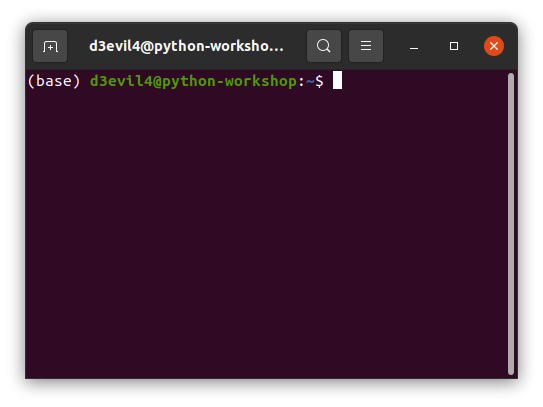
Like Windows, we can type python commands in the Linux terminal to check whether Python is installed or not; if python is installed on your PC, you will see the image below.
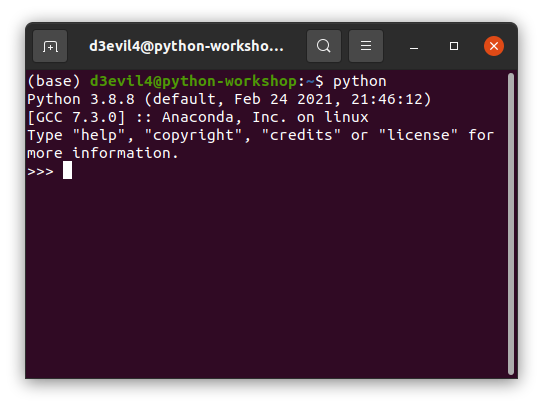
To run a python program on Linux, you can simply use the python command followed by the program name, as shown below.
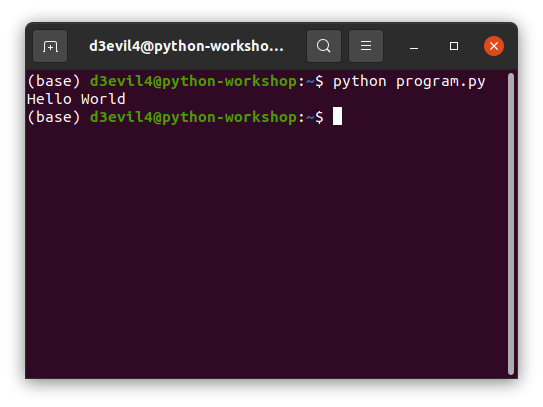
Let see few more examples and run it.
Example: How to average a list in python
To find the average of a list in Python, you can use the built-in sum()
and len()
functions. Here’s an example:
my_list = [10, 20, 30, 40, 50] average = sum(my_list) / len(my_list) print("The average is:", average)
In this example, we first define a list of numbers called my_list
. Then, we use the sum()
function to add up all the numbers in the list and divide the result by the length of the list using the len()
function to get the average. Finally, we print the result using the print()
function.
Output:
The average is: 30.0
Note that the result is a float value, even though the numbers in the list are integers. If you want to round the result to a certain number of decimal places, you can use the built-in round()
function. For example:
my_list = [10, 20, 30, 40, 50] average = round(sum(my_list) / len(my_list), 2) print("The average is:", average)
In this example, we use the round()
function to round the average to 2 decimal places. The second argument of the round()
function specifies the number of decimal places to round to.
Output:
The average is: 30.0
FAQs
Here are some frequently asked questions related to the first Python program:
What is a Python program?
A Python program is a set of instructions that a computer can follow to perform a specific task. Python is a high-level programming language that is easy to learn and use, making it popular for beginners and experienced programmers alike.
How do I write my first Python program?
To write your first Python program, you need to have Python installed on your computer. You can then open a text editor, type your code into a new file, and save the file with a .py extension. You can run the program by using the Python interpreter or an IDE.
What should my first Python program do?
Your first Python program can be simple, such as printing “Hello, World!” to the console. This is a common starting point for beginners, as it teaches the basic syntax of Python and how to run a program.
How do I run a Python program?
To run a Python program, you can use the Python interpreter or an IDE. In the interpreter, you can type “python your_program_name.py” in the command prompt or terminal. In an IDE, you can click the “Run” button or use a keyboard shortcut.
What are some common errors in Python programs?
Common errors in Python programs include syntax errors, indentation errors, and name errors. Syntax errors occur when the code violates the rules of the Python language. Indentation errors occur when the code is not indented properly. Name errors occur when the code refers to a variable or function that has not been defined.
How can I troubleshoot errors in my Python program?
To troubleshoot errors in your Python program, you can use the error messages to identify the source of the problem. You can also use debugging tools in your IDE to step through the code and see where the error occurs. Additionally, you can search online forums and documentation for solutions to common errors.