In this tutorial, we will learn about python int() method and its uses with examples.
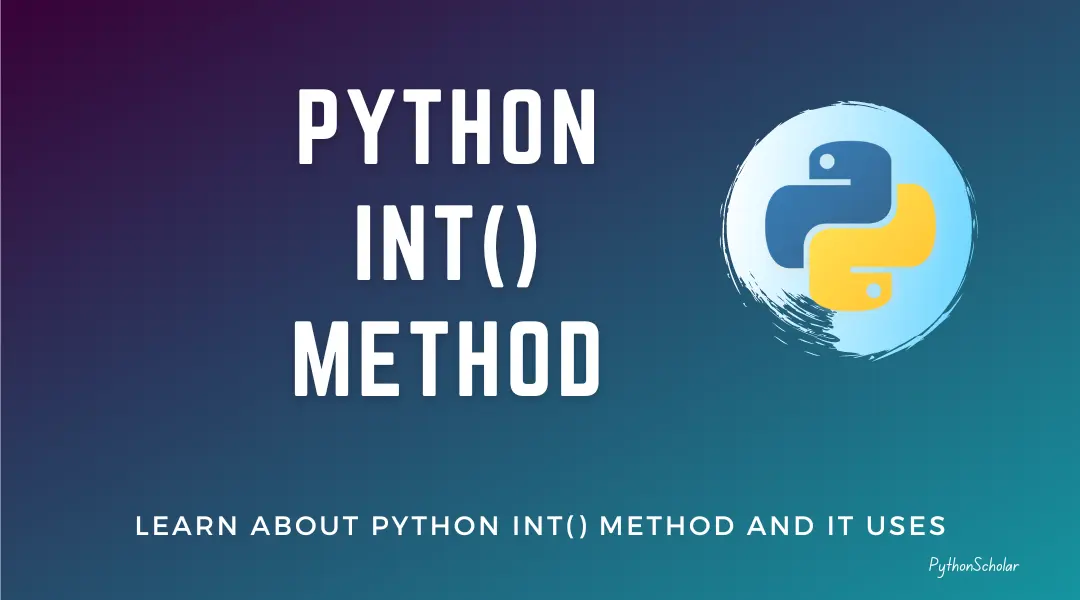
The int() is the built-in method of python, which converts any number or string objects into an integer object; it is also used for typecasting the integer number.
The syntax of int() is:
int(value, base)
int() Parameters
int() method takes two parameters as an argument:
- value – A number or string object which wants to convert into an integer number. By default, it takes zero as an argument.
- base – A base number that represents the number format. The default value is 10.
Let us see some examples of int() methods in python.
Example 1: How to use the int() method in python?
print(int(123))
print(int(123.456))
print(int(“123”))
Output:
123
123
123
Example 2: How int() works for decimal, octal, and hexadecimal?
# binary 0b or 0B
print(“For 1010, int is:”, int(‘1010’, 2))
print(“For 0b1010, int is:”, int(‘0b1010’, 2))
# octal 0o or 0O
print(“For 12, int is:”, int(’12’, 8))
print(“For 0o12, int is:”, int(‘0o12’, 8))
# hexadecimal
print(“For A, int is:”, int(‘A’, 16))
print(“For 0xA, int is:”, int(‘0xA’, 16))
Output:
For 1010, int is: 10
For 0b1010, int is: 10
For 12, int is: 10
For 0o12, int is: 10
For A, int is: 10
For 0xA, int is: 10
It will cause an “invalid literal for int()” error when using strings with non-numerical values.
print(int(“Python”))
The output will be as follow:
Traceback (most recent call last):
File “”, line 1, in <module>
print(int(“Python”))
ValueError: invalid literal for int() with base 10: ‘Python’</module>
Example 3: Taking input as integer and float in input() method.
# Taking input in integer and float
user_Input = int(input(“Enter anything:”))
print(“The inputted value is:”, user_Input)
print(type(user_Input))
user_Input = float(input(“Enter anything:”))
print(“The inputted value is:”, user_Input)
print(type(user_Input))
The output will be as follows.
Enter anything:25
The inputted value is: 25
<class ‘int’=“”>
Enter anything:25
The inputted value is: 25.0
<class ‘float’=“”></class></class>
Rules of int() method
- an integer object from the given number or string treats the default base as 10
- (No parameters) returns 0
- (If base given) treats the string in the given base (0, 2, 8, 10, 16)