Table of Contents
If you use Python to develop data visualisations and user interfaces, you probably know that you have the ability to change the font size of text elements in plots, charts, and other project components. However, you might be unfamiliar with some of the most common ways this is done, such as using Tkinter or Matplotlib. This tutorial aims to provide an introduction to how to change font size in python applications and help dispel any uncertainty around this topic.
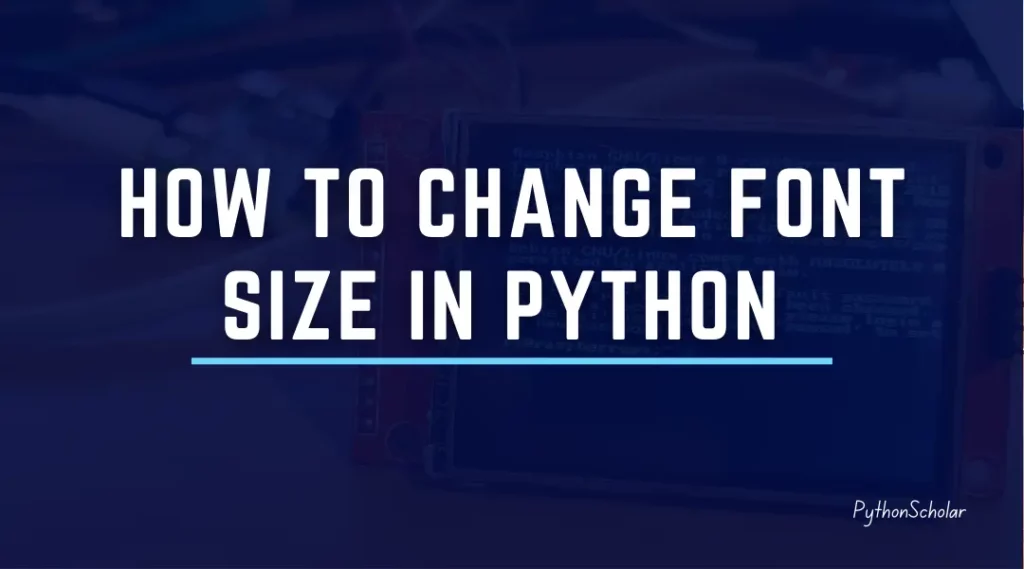
Introduction: Why Changing Font Size Matters in Python
Python is a popular programming language that is widely used for data analysis, machine learning, scientific computing, and more. One important aspect of creating visualisations and user interfaces in Python is the ability to customise the font size of text elements. This is because font size can significantly impact the readability and aesthetic appeal of a project, especially when dealing with large amounts of data or complex interfaces.
Fortunately, Python provides several libraries that allow developers to change the font size of text elements in various contexts. For instance, Matplotlib and Seaborn are popular data visualization libraries that offer extensive customization options for font size in plots and charts. Similarly, Tkinter is a standard GUI toolkit for Python that allows developers to adjust font size and style in graphical user interfaces.
In this blog post, we will explore different ways to change font size in Python using various libraries and techniques. Whether you are a data analyst, machine learning engineer, or GUI developer, this post will help you improve the readability and visual appeal of your Python projects by providing simple and practical examples.
Let’s see some examples of how to change the font size in python
Using Matplotlib: Changing Font Size in Plots and Charts
Matplotlib is a popular data visualization library that provides various tools for creating high-quality plots and charts in Python. One essential aspect of data visualization is the ability to customize font size to improve readability and visual appeal. In this section, we will explore how to change font size in Matplotlib using various techniques.
How to change font size in python Matplotlib
Let’s see some examples of how we can change font size in python matplotlib
Changing Font Size for Entire Plot
To change the font size of all text elements in a plot, you can use the rcParams dictionary. This dictionary contains various default settings for Matplotlib and can be used to set the font size for all text elements in a plot. Here’s an example:
import matplotlib.pyplot as plt # Set font size for all text elements in plot plt.rcParams.update({'font.size': 12}) # Create a plot plt.plot([1, 2, 3], [4, 5, 6]) # Add labels and title plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('My Plot') # Show plot plt.show()
In this example, we set the font size to 12 using the update method of the rcParams dictionary. This changes the font size of all text elements in the plot, including the axis labels and title.
Changing Font Size for Specific Text Elements
Sometimes, you may want to change the font size for specific text elements in a plot, such as the axis labels or legend. Matplotlib provides several methods for achieving this.
a. Changing Font Size for Axis Labels
To change the font size for axis labels, you can use the set_xlabel and set_ylabel methods of the Axes object. Here’s an example:
import matplotlib.pyplot as plt # Create a plot fig, ax = plt.subplots() ax.plot([1, 2, 3], [4, 5, 6]) # Set font size for axis labels ax.set_xlabel('X-axis', fontsize=14) ax.set_ylabel('Y-axis', fontsize=14) # Show plot plt.show()
In this example, we set the font size for both axis labels to 14 using the fontsize argument of the set_xlabel and set_ylabel methods.
b. Changing Font Size for Legend
To change the font size for the legend in a plot, you can use the fontsize argument of the legend method. Here’s an example:
import matplotlib.pyplot as plt # Create a plot with legend fig, ax = plt.subplots() ax.plot([1, 2, 3], [4, 5, 6], label='Line 1') ax.plot([1, 2, 3], [7, 8, 9], label='Line 2') ax.legend(fontsize=12) # Show plot plt.show()
In this example, we set the font size for the legend to 12 using the fontsize argument of the legend method.
Changing font size is an important aspect of creating readable and aesthetically pleasing plots and charts in Python. Matplotlib provides several methods for changing font size, from setting the font size for all text elements in a plot using the rcParams dictionary, to changing the font size for specific text elements such as axis labels and legends. By using these techniques, you can improve the readability and visual appeal of your Matplotlib plots and charts.
Styling Seaborn: Adjusting Font Size in Data Visualizations
Seaborn is a popular data visualization library that builds on top of Matplotlib and provides several high-level functions for creating beautiful and informative statistical graphics in Python. One important aspect of creating visualizations is the ability to adjust font size to improve readability and visual appeal. In this section, we will explore how to adjust font size in Seaborn using various techniques.
How to change font size in python Seaborn
Let’s see some examples of how we can change font size in python seaborn
Changing Font Size for Entire Plot
To change the font size for all text elements in a Seaborn plot, you can use the set method of the rc object. This object provides a simple interface to customize various settings for Seaborn plots, including font size. Here’s an example:
import seaborn as sns # Set font size for all text elements in plot sns.set(font_scale=1.2) # Create a plot tips = sns.load_dataset("tips") sns.scatterplot(x="total_bill", y="tip", data=tips) # Show plot sns.plt.show()
In this example, we set the font size for all text elements in the plot using the set method of the rc object. This changes the font size of all text elements in the plot, including the axis labels and legend.
Changing Font Size for Specific Text Elements
Sometimes, you may want to change the font size for specific text elements in a Seaborn plot, such as the axis labels or legend. Seaborn provides several functions for achieving this.
a. Changing Font Size for Axis Labels
To change the font size for axis labels in a Seaborn plot, you can use the set_xlabel and set_ylabel methods of the Axes object. Here’s an example:
import seaborn as sns # Create a plot with axis labels tips = sns.load_dataset("tips") ax = sns.scatterplot(x="total_bill", y="tip", data=tips) ax.set_xlabel("Total Bill", fontsize=14) ax.set_ylabel("Tip Amount", fontsize=14) # Show plot sns.plt.show()
In this example, we set the font size for both axis labels to 14 using the fontsize argument of the set_xlabel and set_ylabel methods.
b. Changing Font Size for Legend
To change the font size for the legend in a Seaborn plot, you can use the fontsize argument of the legend method. Here’s an example:
import seaborn as sns # Create a plot with legend tips = sns.load_dataset("tips") ax = sns.scatterplot(x="total_bill", y="tip", hue="day", data=tips) ax.legend(fontsize=12) # Show plot sns.plt.show()
In this example, we set the font size for the legend to 12 using the fontsize argument of the legend method.
Changing font size is an important aspect of creating readable and aesthetically pleasing data visualizations in Seaborn. Seaborn provides several methods for changing font size, from setting the font scale for all text elements in a plot using the set method of the rc object, to changing the font size for specific text elements such as axis labels and legends using various functions and methods. By using these techniques, you can improve the readability and visual appeal of your Seaborn plots and create more informative data visualizations.
Creating GUIs with Tkinter: Customizing Text Font Size and Style
Tkinter is a standard Python library for creating graphical user interfaces (GUIs). It provides a simple way to create windows, buttons, labels, and other graphical elements. One important aspect of creating GUIs is customizing the text font size and style. In this section, we will explore how to customize the font size and style in Tkinter.
How to change font size in python tkinter
Let’s see some examples of how we can change font size in python tkinter
Changing Font Size
To change the font size of text in Tkinter, you can use the font parameter when creating a text widget or label. The font parameter takes a tuple of two elements: the font family and font size. Here’s an example:
import tkinter as tk # Create a text widget with custom font size root = tk.Tk() text_widget = tk.Text(root, font=("Arial", 14)) text_widget.pack() # Start GUI root.mainloop()
In this example, we create a text widget with a font size of 14 points and the Arial font family. You can change the font size to any value that suits your needs.
Changing Font Style
To change the font style of text in Tkinter, you can use the font parameter with additional arguments to specify the font style, such as bold or italic. Here’s an example:
import tkinter as tk # Create a label with custom font style root = tk.Tk() label = tk.Label(root, text="Hello, world!", font=("Arial", 14, "bold")) label.pack() # Start GUI root.mainloop()
In this example, we create a label with a font size of 14 points, the Arial font family, and the bold font style. You can also use other font styles such as italic or underline.
Changing Default Font
To change the default font for all widgets in Tkinter, you can use the font parameter with the tkinter.font module. This module provides a way to create font objects with various properties such as font family, font size, and font style. Here's an example: import tkinter as tk import tkinter.font as tkfont # Create a custom font object custom_font = tkfont.Font(family="Arial", size=14) # Set the default font for all widgets root = tk.Tk() root.option_add("*Font", custom_font) # Create a label with default font label = tk.Label(root, text="Hello, world!") label.pack() # Start GUI root.mainloop()
In this example, we create a custom font object with the Arial font family and a font size of 14 points. We then set the default font for all widgets using the option_add method of the root window. Finally, we create a label with the default font.
Customizing the font size and style is an important aspect of creating GUIs in Tkinter. Tkinter provides several ways to change the font size and style, from using the font parameter when creating widgets to setting the default font for all widgets using the tkinter.font module. By using these techniques, you can create GUIs that are more readable and visually appealing to users.
Python Interpreter: Changing Font Size in Terminal and IDEs
The Python interpreter is the command-line tool that allows you to execute Python code. When working with the interpreter, it’s important to have a font size that’s easy to read. In addition to the interpreter, most Python Integrated Development Environments (IDEs) also provide ways to change the font size. In this section, we will explore how to change the font size in the Python interpreter and some popular IDEs.
How to change font size in python idle
Let’s see some examples of how we can change font size in python idle
Python Interpreter
Most terminal emulators allow you to change the font size using a keyboard shortcut. For example, in the macOS Terminal, you can use the Command + Plus or Command + Minus keyboard shortcuts to increase or decrease the font size, respectively. In the Windows Command Prompt, you can use the Control + Mouse Scroll Wheel to change the font size.
You can also change the font size permanently in the terminal emulator settings. For example, in the macOS Terminal, you can go to Terminal > Preferences > Profiles > Text and adjust the font size. In the Windows Command Prompt, you can right-click on the title bar and go to Properties > Font.
PyCharm
PyCharm is a popular Python IDE that provides a variety of ways to customize the font size. You can adjust the font size of the editor, console, and other UI elements.
To change the font size in PyCharm, go to File > Settings > Editor > Font. Here, you can adjust the font size for the editor and other UI elements. You can also change the font family and style.
Visual Studio Code
Visual Studio Code is another popular Python IDE that provides a variety of ways to customize the font size. You can adjust the font size of the editor, terminal, and other UI elements.
To change the font size in Visual Studio Code, go to File > Preferences > Settings. Here, you can adjust the font size for the editor and other UI elements. You can also change the font family and style.
Changing the font size in the Python interpreter and IDEs is essential for a comfortable and efficient coding experience. Whether you’re working in the terminal or an IDE, there are always ways to customize the font size to your liking. By using the techniques outlined in this section, you can optimize your coding environment and work more efficiently.
How to change font size in python idle in windows
To change the font size in Python IDLE in Windows, follow these steps:
- Open Python IDLE.
- Go to Options > Configure IDLE.
- In the IDLE Preferences window, select the Fonts/Tabs tab.
- In the Fonts section, you can select the font family, font size, and style for each of the following elements:
- Normal text
- Calltips
- Output
- Shell
- Select the element you want to change the font size for, and adjust the font size using the Size dropdown menu.
- Click the OK button to save your changes and close the IDLE Preferences window.
Your selected font size will now be applied to the corresponding element in Python IDLE.
Conclusion: Best Practices for Changing Font Size in Python
Changing the font size is an important aspect of customizing the appearance of your Python code and data visualizations. By following best practices, you can optimize your coding environment and ensure that your code is easy to read and understand. Here are some key takeaways to keep in mind when changing font size in Python:
- Use a consistent font size across your code, data visualizations, and documentation to ensure a cohesive look and feel.
- When working with data visualizations, make sure that the font size is large enough to be legible, but not so large that it detracts from the data itself.
- When working with text-based interfaces like the Python interpreter and IDEs, adjust the font size to a level that is comfortable for you to read and work with.
- Take advantage of the customization options provided by libraries like Matplotlib, Seaborn, and Tkinter to adjust font size in data visualizations and GUIs.
- Consider the readability of your code when adjusting font size. Avoid using font sizes that are too small or too large, as this can make your code harder to read and understand.
By following these best practices, you can ensure that your Python code and data visualizations are not only functional but also visually appealing and easy to work with. Remember, a well-designed and readable codebase can make all the difference when it comes to collaborating with others and maintaining your code over time.
FAQS on How to Change Font Size in Python
Can I change the font size of text printed in the console?
Yes, you can change the font size of text printed in the console by adjusting the font size settings in your terminal emulator.
How do I change the font size in Matplotlib plots?
You can change the font size of text in Matplotlib plots by setting the font size parameter in the plot function, or by using the rcParams dictionary to set the font size globally.
How can I change the font size of text in Seaborn data visualizations?
You can change the font size of text in Seaborn data visualizations by using the set() function to set the font scale, or by setting the font size parameter in individual plot functions.
How do I change the font size in Tkinter GUIs?
You can change the font size in Tkinter GUIs by setting the font parameter for individual widgets, or by using the configure() method to set the font globally for all widgets.
Can I change the font size in Python IDEs like PyCharm and Visual Studio Code?
Yes, you can change the font size in Python IDEs like PyCharm and Visual Studio Code by adjusting the font size settings in the preferences or settings menu.
Is it important to consider font size when writing Python code?
Yes, font size is an important aspect of code readability and can have a significant impact on how easy it is to understand and maintain your code. It’s important to choose a font size that is comfortable to read and consistent across your codebase.
Related Python Projects
How to make a Hangman Game in Python – [GUI Source Code]
How to Make a Rule based Chatbot in Python using Flask
Convert Celsius to Fahrenheit in Python – [With Chart]