In this tutorial, we will build Bank Management System Project in Python. In today’s world, managing a bank and its customers’ accounts manually can be a daunting task. Therefore, automating the process can improve efficiency and reduce errors. This is where a Bank Management System comes in handy.
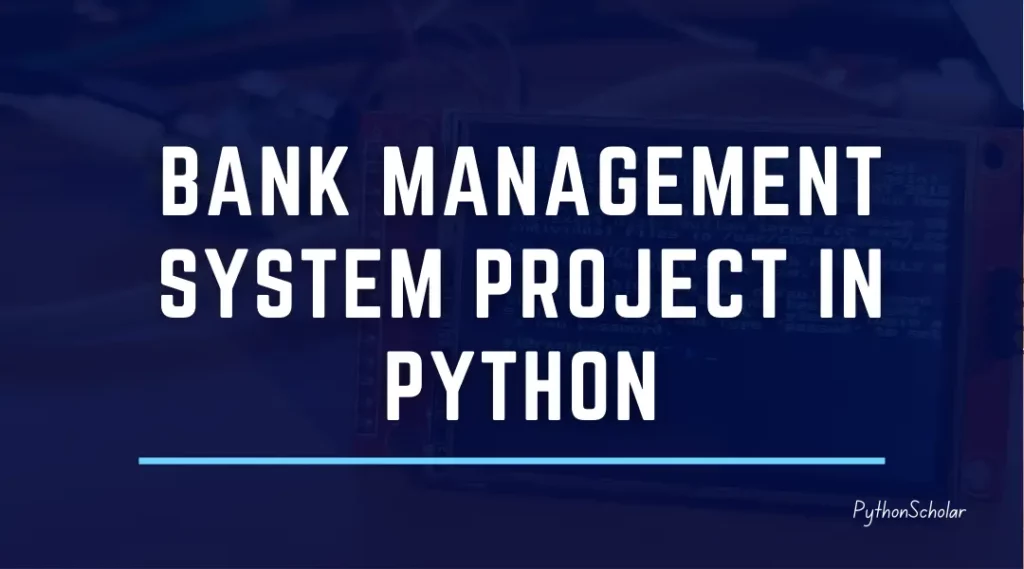
This project is developed using Python, a popular programming language known for its simplicity and ease of use. With the Bank Management System project in Python, you can manage customer accounts, process transactions, generate reports, and much more.
This project is not only useful for banks but it can be used for learners to build a bank management college project. The system provides a user-friendly interface that enables users to perform transactions easily and quickly.
Overall, this Bank Management System project in Python is a practical and valuable tool for anyone looking to streamline their financial operations.
What is Bank Management System?
A Bank Management System is a software application that helps banks and financial institutions manage their operations and transactions. It is designed to automate banking processes and provide a platform for customers to access their accounts and perform transactions online.
The system provides various features such as customer account management, transaction processing, balance checking, account statements, fund transfers, bill payments, loan management, and more. Bank Management Systems also offer features for bank employees to manage customer data, process transactions, and generate reports.
By using a Bank Management System, banks can streamline their operations and reduce the workload of their employees. Customers can also access their accounts and perform transactions from the comfort of their homes or offices, reducing the need to physically visit the bank. The system also provides a secure platform for transactions, ensuring that customers’ personal and financial information is kept safe.
Project Prerequisites:
In this python project, you just need to know basic python. That includes.
What will you learn?
- End to End development of python project.
- Basic Input / Output implementation.
Outlines of the Project:
- Define the required classes
- Implement the user interface
- Implement the logic
- Test the system
How to make Bank Management System Project in Python
Let’s start developing a bank management system project in python step by step.
Define the required classes
You will need to create a few classes for this project, such as a Bank class, Account class, and Transaction class.
- Bank: contains a list of accounts and methods to add and remove accounts.
- Account: contains the account number, owner name, balance, and methods to deposit, withdraw, and get the balance.
- Transaction: contains the transaction amount and type (deposit or withdrawal).
Bank class:
- Properties: name, accounts (list)
- Methods:
- init(self, name): initializes the Bank object with a given name and an empty list of accounts.
- add_account(self, account): adds an Account object to the accounts list.
- remove_account(self, account): removes an Account object from the accounts list.
class Bank: def __init__(self, name): self.name = name self.accounts = [] def add_account(self, account): self.accounts.append(account)
Account class:
- Properties: number, owner, balance, transactions (list)
- Methods:
- init(self, number, owner, balance): initializes the Account object with a given account number, owner name, and balance.
- deposit(self, amount): adds the amount to the balance and appends a Transaction object to the transactions list with the type ‘Deposit’.
- withdraw(self, amount): subtracts the amount from the balance if there are sufficient funds and appends a Transaction object to the transactions list with the type ‘Withdrawal’.
- get_balance(self): returns the current balance of the account.
class Account: def __init__(self, number, owner, balance): self.number = number self.owner = owner self.balance = balance self.transactions = [] def deposit(self, amount): self.balance += amount self.transactions.append(Transaction(amount, 'Deposit')) def withdraw(self, amount): if self.balance >= amount: self.balance -= amount self.transactions.append(Transaction(amount, 'Withdrawal')) else: print('Insufficient funds')
Transaction class:
- Properties: amount, type
- Methods:
- init(self, amount, type): initializes the Transaction object with a given amount and type.
class Transaction: def __init__(self, amount, type): self.amount = amount self.type = type
Implement the user interface
Next, you’ll need to create a user interface for your Bank Management System.
Here’s an outline for creating a user interface in a Bank Management System project in Python:
- Welcome message: display a welcome message to the user and prompt them to choose an option.
- Options menu: display a menu with the following options:
- Create account: prompts the user to enter the account number, owner name, and initial balance.
- Deposit: prompts the user to enter the account number and deposit amount.
- Withdraw: prompts the user to enter the account number and withdrawal amount.
- Check balance: prompts the user to enter the account number and displays the current balance.
- Transaction history: prompts the user to enter the account number and displays a list of transactions for the account.
- Quit: exits the program.
- User input: use the input() function to get the user’s choice and input data.
- Data validation: use conditionals to check the validity of the user’s input, such as whether the account number exists, the deposit amount is positive, or the withdrawal amount does not exceed the account balance.
- Output: use the print() function to display the results of the user’s actions, such as the new account balance or the transaction history.
- Loop: use a loop to repeat the options menu until the user chooses to quit.
Implement the logic
Next, you’ll need to create a user interface for your Bank Management System.
- Initialize the Bank object with a name and an empty list of accounts.
- Use a loop to display the options menu and get the user’s choice.
- Depending on the user’s choice, perform the corresponding action using conditionals:
- Create account: create a new Account object with the given account number, owner name, and balance, and add it to the accounts list of the Bank object.
- Deposit: search for the Account object with the given account number and call its deposit() method with the given amount.
- Withdraw: search for the Account object with the given account number and call its withdraw() method with the given amount.
- Check balance: search for the Account object with the given account number and call its get_balance() method to display the current balance.
- Transaction history: search for the Account object with the given account number and display the list of transactions stored in its transactions property.
- Quit: exit the program.
- Use loops and conditionals to search for Account objects with the given account number, and check their validity before performing transactions. For example, if the account number is not found, display an error message.
- Use lists to store the transaction history for each Account object, and update it whenever a deposit or withdrawal is made.
def main(): bank_name = input('Enter bank name: ') bank = Bank(bank_name) print('Bank', bank_name, 'created successfully') while True: print('1. Create Account') print('2. Deposit') print('3. Withdraw') print('4. Exit') choice = int(input('Enter your choice: ')) if choice == 1: acc_number = input('Enter account number: ') acc_owner = input('Enter account owner name: ') acc_balance = float(input('Enter opening balance: ')) account = Account(acc_number, acc_owner, acc_balance) bank.add_account(account) print('Account created successfully') elif choice == 2: acc_number = input('Enter account number: ') amount = float(input('Enter amount to deposit: ')) account = find_account(bank.accounts, acc_number) if account: account.deposit(amount) print('Deposit successful') else: print('Account not found') elif choice == 3: acc_number = input('Enter account number: ') amount = float(input('Enter amount to withdraw: ')) account = find_account(bank.accounts, acc_number) if account: account.withdraw(amount) print('Withdrawal successful') else: print('Account not found') elif choice == 4: print('Thank you for using the Bank Management System') break else: print('Invalid choice') def find_account(accounts, number): for account in accounts: if account.number == number: return account return None if __name__ == '__main__': main()
Test the system:
Now that you have the classes and user interface implemented, you can test the Bank Management System. Here’s an example of how you can create an account, deposit money, and withdraw money:
Testing is an important part of software development, including for a Bank Management System project in Python. After defining the classes and implementing the logic, it is essential to test the system to ensure that it works as expected and meets the requirements.
Testing can involve a variety of methods, such as unit testing, integration testing, and acceptance testing. Unit testing involves testing individual components of the system, such as classes and methods, to ensure that they work correctly. Integration testing involves testing how different components of the system work together to ensure that they integrate properly. Acceptance testing involves testing the system as a whole to ensure that it meets the requirements and expectations of the users.
In a Bank Management System project in Python, testing can involve creating test cases for each of the system’s features, such as creating an account, making a deposit, withdrawing funds, checking the balance, and viewing the transaction history. Test cases can include both valid and invalid inputs to ensure that the system handles errors and exceptions properly.
Output:
Enter bank name: ABC Bank Bank ABC Bank created successfully 1. Create Account 2. Deposit 3. Withdraw 4. Exit Enter your choice: 1 Enter account number: 1234 Enter account owner name: John Doe Enter opening balance: 5000 Account created successfully 1. Create Account 2. Deposit 3. Withdraw 4. Exit Enter your choice: 2 Enter account number: 1234 Enter amount to deposit:
Download bank management system projection python source code
Conclusion on bank management system project in python
In conclusion, a Bank Management System project in Python can be a useful tool for managing banking operations and transactions. By defining classes and implementing the logic, the system can provide various features such as creating and managing accounts, depositing and withdrawing funds, checking balances, and viewing transaction history.
FAQs
What is a Bank Management System project in Python?
A Bank Management System project in Python is a software application that allows users to perform banking transactions such as creating and managing accounts, depositing and withdrawing funds, checking balances, and viewing transaction history.
What are the benefits of developing a Bank Management System project in Python?
Developing a Bank Management System project in Python provides practical experience in object-oriented programming, data structures, algorithms, and software development practices. It can also be customized to meet specific business needs and requirements.
What are the basic requirements for developing a Bank Management System project in Python?
The basic requirements for developing a Bank Management System project in Python include defining classes, implementing the logic, creating a user interface, and testing the system.
What tools and libraries can be used for creating a user interface in a Bank Management System project in Python?
Various tools and libraries can be used for creating a user interface in a Bank Management System project in Python, such as tkinter, PyQt, or wxPython.
What testing methods can be used for testing a Bank Management System project in Python?
Various testing methods can be used for testing a Bank Management System project in Python, such as unit testing, integration testing, and acceptance testing.
Can a Bank Management System project in Python be used in real-life banking operations?
Yes, a Bank Management System project in Python can be used in real-life banking operations. However, it is important to ensure that the system meets regulatory and security requirements and is thoroughly tested before deployment.
How can a Bank Management System project in Python be extended or customized to meet specific business needs?
A Bank Management System project in Python can be extended or customized by adding new features, such as interest calculations, loan management, or online banking services, or by integrating with other systems and services.
List of Other Python Projects
How to make a Hangman Game in Python – [GUI Source Code]
How to Make a Rule based Chatbot in Python using Flask
Convert Celsius to Fahrenheit in Python – [With Chart]