In this blog, we will learn what the Langchain framework is and how it is used to develop LLM applications. This framework enables the addition of ChatGPT with memory and ChatGPT long-term memory, along with prompts.
Let understand what langchain is and what are langchain features and uses
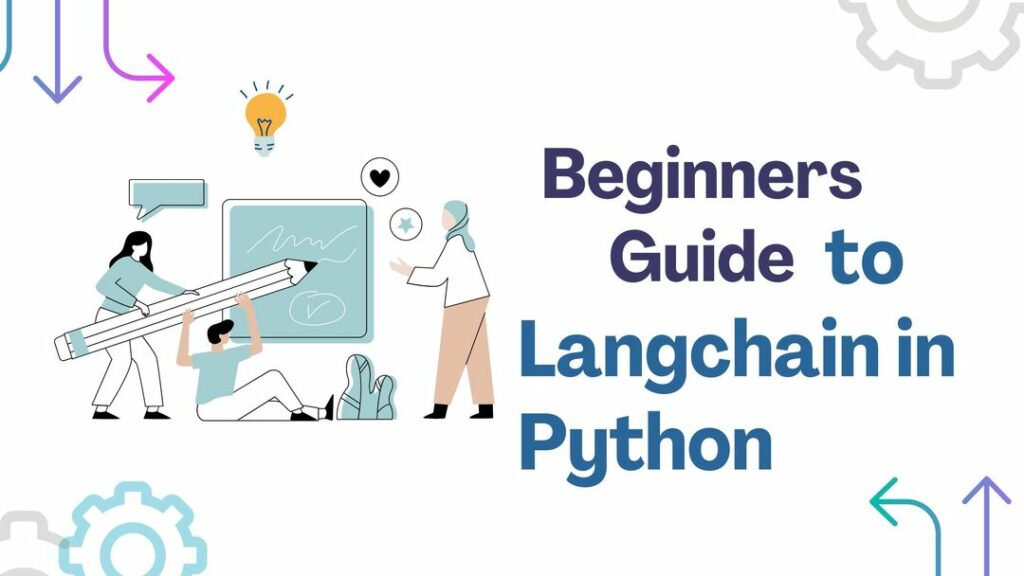
What is LangChain?
LangChain is a python framework designed to simplify the creation of AI based python applications using large language models (LLMs). Langchain consists of two words, lang+chain, where lang stands for language and chain means as it says a chain.
Why use Langchain?
Here are some of the benefits of using LangChain:
- Ease of use: LangChain makes it easy to create applications using LLMs. The framework provides a standard chain interface, making connecting different LLMs and other tools easy.
- Flexibility: LangChain is a flexible framework that can be used for various applications. The framework provides multiple tools and integrations so that you can choose the right tools for your specific application.
- Community: LangChain has a large and active community of developers. This community provides support and resources for LangChain users.
Who is this guide for?
One who is working with LLM models, a student who wants to learn about large language models, is for someone who wants to develop excellent NLP-backed applications.
Now it is time to play around with langchain. Let’s start coding now.
Langchain Installation and setup
First, we will set up a python virtual environment and install the necessary libraries.
Setting up your development environment
Follow the below step to set up the Python virtual environment.
Step 1: Use the necessary command to create the environment.
python -m venv envname |
Step 2: Active virtual environment.
Windows:
\venvname\Scripts\activate |
Linux & Mac
source /venvname/bin/activate
Once our environment is activated, we will install the required packages or libraries we need for our projects.
Step 3: Now we install the langchain framework in our python environment.
pip install langchain |
If you face a no module named ‘langchain’ error, double check the python version or check if langchain is installed successfully.
You can learn more about how to use ChatGPT using python from here.
Features of Langchain
Below are the fantastic features that come with langchain
Models I/O
The central core elements of any application are the model or model input and out. And langchain has features or elements like prompts, language model(large language model) and output parsers.
Let us understand one by one.
Prompts
Prompts serve as a way to guide the language model’s responses and make them more valuable and relevant to a specific task or application. They help users to obtain more targeted and coherent outputs from the model.
Language Models
In langchain, we can use two types of language models LLMs and chat models. The LLMs models are simple large language models that take input as a string and return the string. Were’s, on the other hand, chat models that series of chat messages and return a chat response.
Output Parsers
Output parsers are simply classes that help LLM models for structure responses.
Chains
Chains allow us to integrate multiple components seamlessly, forming a unified and coherent application.
For instance, we can construct a chain that takes user input, applies a PromptTemplate to format it appropriately, and subsequently feeds the formatted output into an LLM (Language Model). We can create even more intricate and sophisticated chains by merging various chains or combining them with other components.
Memory
Large language models come with goldfish memory means its models cannot remember the previous conversions or inputs. For example, chatbot applications will forget about past interactions. That’s where langchain’s memory class comes into the picture. It will store all past interactions and send every time with new inputs so that models can recall previous chats.
Using this, we can give LLM modes short-term memory and langchain long-term memory that can help to develop a memory GPT application.
Agents
The agents are the middleware that will direct the chain and choose a sequence of tools to take action. Langchain agents come with many tools that help to create LLM applications.
As our essential is clear about the features of lanchain, let us create an LLM application using langchain in python.
Compare to ChatGPT Google bard AI is also a good option to try with that can be found here Google Bard in Python – How to Use Bard and Lamda.
Creating your first LLM application in Python
Let now step through step process to develop our LLM application.
Step 1: Import required langchain methods.
from langchain import PromptTemplate, LLMChain, OpenAI from langchain.memory import ConversationBufferMemory |
Step 2: We will design a prompt that will give a persona or guidance to the LLM model.
prompt_template = “””You are a chatbot having a conversation with a human. {chat_history} Human: {human_input} Chatbot:””” |
The first line consists of a simple line for a normal chatbot, but you can use techniques like prompt engineering to design a prompt, and the {chat_history} is the prefix for chat history or memory of the LLM model, and the {human_input} is the prefix of the user’s input.
Step 3: Now, we will implement the LLM application’s memory model.
memory = ConversationBufferMemory(memory_key=”chat_history”) |
Step 4: Then, we will use the prompt we design in Step 2 with the PromptTemplate().
prompt=PromptTemplate(input_variables=[“chat_history”, “human_input”], template=prompt_template) |
Step 5: We will now create an llm chain using the LLMChain() method.
llm_chain = LLMChain( llm=OpenAI(), prompt=prompt, verbose=True, memory=memory, ) |
Step 6: Our application is ready to run and follow the below steps to get the responses from our LLM application
print(llm_chain.run(“Who was the 5th president of the united states?”)) |
The output will be as follow:
James Madison was the 5th President of the United States |
As we can see, our large language model is responding like a tremendous artificial intelligence. You can follow the same steps by tweaking the prompt and playing around with langchain memories and agents.
Where to go from here?
As we have sufficient knowledge of langchain, you can follow the additional resources below for more experiments and find langchain examples.
Additional resources
- Langchain documentation: You can follow official langchain python docs from here.
- Langchain tutorials: You can follow youtube and the official langchain website, which has many different tutorials on langchain. Also, blog langchain dev is a fantastic website for langchain developers.
- Langchain community: Langchain has a fantastic community on the langchain github repository page and Reddit.
FAQ’s about LangChain
What is LangChain used for?
LangChain can be used for various applications, including Chatbots, Document summarisation, Code analysis, Question answering, Natural language generation, Text classification and Sentiment analysis.
What is the difference between LangChain and LLM?
The core difference between langchain and LLM is that langchain is a python framework, and the LLM is the large language model or an AI model used for NLP tasks.
Why is it called LangChain?
The reason behind the word langchain is that langchain uses the chain method to develop the LLM model, which is called the large language model; that’s why it’s called langchain.
What version of Python for LangChain?
Most subtable python versions will be 3.7 to 3.11 for langchain use.