In this tutorial, we will explore various ways to replace spaces with underscore using Python programming.
Method 1 – Replace space with underscore in Python using the replace() method.
Here we will use the python replace() method that comes pre-built with python programming and is used to minupliute python string.
Step 1: First we will declar a variable and store a string.
my_string = “I love python programming language.” |
Step 2: We will use the replace() method to replace whitespace with an underscore.
my_string = my_string.replace(‘ ‘,’_’) |
Step 3: Now we will print our results.
print(my_string) |
The Output will be as follow:
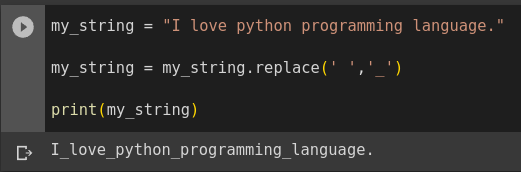
Method 2 – Replace space with underscore in Python using the split() and join() method.
In this second method, we will use two python method that is used to split the string in python and other is join() method is used to join two or more thing together.
Step 1: We will store a string in a python variable.
some_string = “Some sample text for example.” |
Step 2: Then use the split method to split entire text based on whitespace.
some_string = some_string.split() |
Step 3: Now join all text with the join() method.
some_string = ‘_’.join(some_string) |
Step 4: Print the result.
print(some_string) |
Output:
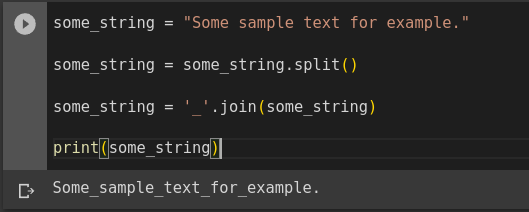
Method 3 – Replace space with underscore in Python using the for loop method.
In the third example, we will use legandy python for loop to replace space with underscore.
Step 1: Let’s first create a variable
string_sample = “This text will replace whitespace with underscore” |
Step 2: We will implement a for loop along with an if condition that will split the text.
string_list = [“_” if x == ” ” else x for x in string_sample] |
Step 3: Join all the split text into one.
string_sample = “”.join(string_list) |
Step 4: Print the results.
Output:
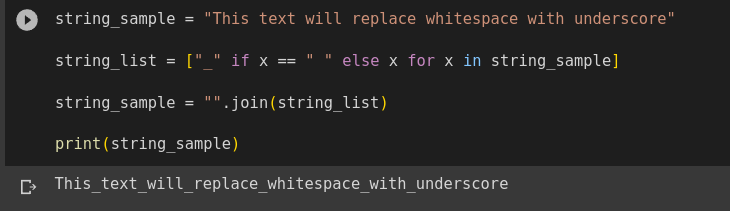
Method 4 – Replace space with underscore in Python using the the re.sub() method.
In this example, we use the power tool called regular expressions function in python to replace spaces with underscore.
Here we will use the re.sub() method. The re.sub() method allows you to substitute occurrences of a pattern with a specified replacement.
Step 1: Import the regular expression function.
import re |
Step 2: Create a variable.
my_string = “Replaceing space with underscore with regular expressions function” |
Step 3: Implement the re.sub() function.
result = re.sub(‘\s+’, ‘_’, my_string) |
In the code above, the regular expression pattern r’\s’ is used to match any whitespace character (spaces, tabs, or newlines). The replacement string replacement = ‘_’ is used to replace each match with an underscore.
The re.sub() function then performs the replacement operation and returns the modified string with spaces replaced by underscores.
Step 4: Print the result.
print(result) |
Output:
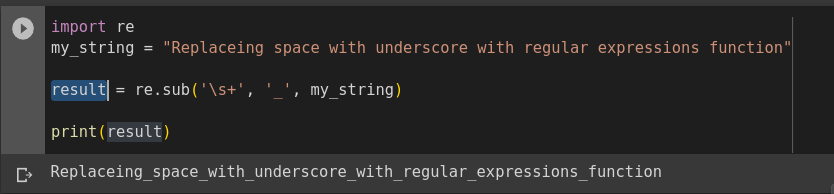
Method 5 – Replace space with underscore in Python using the pythonscholar-replace-space package method
In this example, we will use the pythonscholar-replace-space python package that is used to replace space with an underscore.
First, we will install the pythonscholar-replace-space package by following below step.
pip install pythonscholar-replace-space |
Step 1: Import the required package.
from pythonscholar_replace_space import replace_spaces_with_underscore |
Step 2: Declare a python string.
input_string = “Hello World! This is a test string.” |
Step 3: Implement the replace_spaces_with_underscore function.
output_string = replace_spaces_with_underscore(input_string) |
Step 4: Print the result.
print(output_string) |
Output:
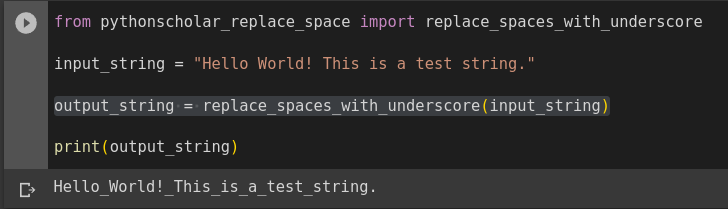
Conclusion
In this tutorial, we go through the different methods in python that are used to replace space with underscore in python string. We use the replace() method, the combination of split() and join() method, we also use for loop and if condition together, we use regular expressions with re.sub(), and an external package called pythonscholar-replace-space. This method can have different ways of implementation but the outcome will be the same.
We hope you like this tutorial and you can use this method for your projects and works.